The following code example will produce two interactive figures. The first one demonstrates how to overplot curves, the second one shows how to get two separate plots on the figure.
#First, import the libraries as plt and np
import matplotlib.pyplot as plt
import numpy as np
#Define a function
def f(t):
return np.exp(-t)*np.cos(2*np.pi*t)
# Set up two arrays
t = np.arange(0.0, 5.0, 0.1)
t2 = np.arange(0.0, 5.0, 0.02)
#make single plot with overplots
plt.plot(t, f(t), 'g^', t, f(t*t), 'r--', t, f(t*t*t), 'bs')
plt.plot(t2, f(t2), 'g', t2, f(t2*t2), 'r', t2, f(t2*t2*t2), 'b')
# add labels
plt.ylabel('y values')
plt.xlabel('x values')
# Example of how to get math characters.
# Not that the format is the same as TeX markup but you don't need to have
# TeX installed since matplotlib has it's own parser, layout engine and fonts.
# For example, to print the greek letter sigma as the x title, use:
# plt.xlabel(r'$\sigma$')
plt.title(r'$\Delta\chi^2$')
# add some text on the plot at location 2, 0.6
plt.text(2, 0.6, r'$\alpha_i=100,\ \Delta\chi^2=15$')
# use gridmarks
plt.grid(True)
#make second plot with 2 subplots
plt.figure(2)
#first subplot
plt.subplot(211)
plt.plot(t, f(t), 'bo', t2, f(t2), 'k')
plt.ylabel('y values')
plt.xlabel('x values')
plt.title('blah')
plt.grid(True)
#second subplot
plt.subplot(212)
plt.plot(t2,np.cos(2*np.pi*t2), 'r--')
plt.ylabel('y2 Values')
plt.xlabel('x2 values')
plt.grid(True)
# realise plot
plt.show()
This snippet will produce the following figures:
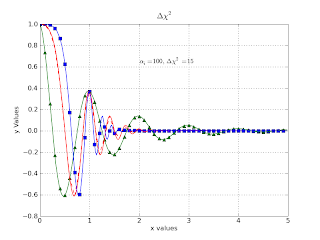
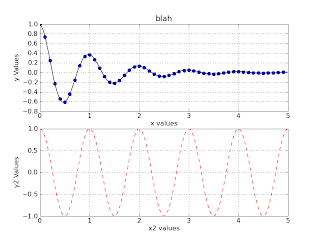
No comments:
Post a Comment